AutoConnectElements
The elements for the custom Web pages¶
Representative HTML elements for making the custom Web page are provided as AutoConnectElements.
- AutoConnectButton: Labeled action button
- AutoConnectCheckbox: Labeled checkbox
- AutoConnectElement: General tag
- AutoConnectFile: File uploader
- AutoConnectInput: Labeled text input box
- AutoConnectRadio: Labeled radio button
- AutoConnectRange: Labeled range slider
- AutoConnectSelect: Selection list
- AutoConnectStyle: Custom CSS code
- AutoConnectSubmit: Submit button
- AutoConnectText: Style attributed text
Layout on a custom Web page¶
AutoConnect will not actively be involved in the layout of custom Web pages generated from AutoConnectElements. However, each element has an attribute to arrange placement on a custom web page by horizontally or vertically.
Custom CSS for a custom Web page¶
All custom Web page styles are limited to the built-in unique CSS embedded in the library code. Direct modification of the CSS affects AutoConnect behavior. You can use dedicated elements to relatively safely modify the style of your custom Web page. The AutoConnectStyle will insert the raw CSS code into the style block in HTML of the custom Web page.
Form and AutoConnectElements¶
All AutoConnectElements placed on custom web pages will be contained into one form. Its form is fixed and created by AutoConnect. The form value (usually the text or checkbox you entered) is sent by AutoConnectSubmit using the POST method with HTTP. The post method sends the actual form data which is a query string whose contents are the name and value of AutoConnectElements. You can retrieve the value for the parameter with the Sketch from the query string with ESP8266WebServer::arg function or PageArgument class of the AutoConnect::on handler when the form is submitted.
AutoConnectElement - A basic class of elements¶
AutoConnectElement is a base class for other element classes and has common attributes for all elements. It can also be used as a variant of each element. The following items are attributes that AutoConnectElement has and are common to other elements.
Sample
AutoConnectElement element("element", "<hr>");
On the page:
Constructor¶
AutoConnectElement(const char* name, const char* value, const ACPosterior_t post)
name¶
Each element has a name. The name is the String data type. You can identify each element by the name to access it with sketches.
value¶
The value is the string which is a source to generate an HTML code. Characteristics of Value vary depending on the element. The value of AutoConnectElement is native HTML code. A string of value is output as HTML as it is.
post¶
The post specifies a tag to add behind the HTML code generated from the element. Its purpose is to place elements on the custom Web page as intended by the user sketch. AutoConnect will not actively be involved in the layout of custom Web pages generated from AutoConnectElements. Each element follows behind the previous one, with the exception of some elements. You can use the post value to arrange vertically or horizontal when the elements do not have the intended position on the custom Web Page specifying the following enumeration value as ACPosterior_t type for the post.
AC_Tag_None
: No generate additional tags.AC_Tag_BR
: Add a<br>
tag to the end of the element.AC_Tag_P
: Include the element in the<p> ~ </p>
tag.AC_Tag_DIV
: Include the element in the<div> ~ </div>
tag.
The default interpretation of the post value is specific to each element.
AutoConnectElements | Default interpretation of the post value |
---|---|
AutoConnectElement | AC_Tag_None |
AutoConnectButton | AC_Tag_None |
AutoConnectCheckBox | AC_Tag_BR |
AutoConnectFile | AC_Tag_BR |
AutoConnectInput | AC_Tag_BR |
AutoConnectRadio | AC_Tag_BR |
AutoConnectRange | AC_Tag_BR |
AutoConnectSelect | AC_Tag_BR |
AutoConnectSubmit | AC_Tag_None |
AutoConnectText | AC_Tag_None |
A placement posterior of AutoConnectText
A placement posterior for AutoConnectText has a slightly peculiar specification. AutoConnectText element without the style attribute will be drained to HTML as a raw value and is accompanied by <p>
, <br>
or <div>
tags according to the post enumeration values.
If the style attribute is specified, the post enumeration value will be ignored and always be enclosed in the <div>
tag, and the style value will be inserted into style
attribute of the <div>
tag.
type¶
The type indicates the type of the element and represented as the ACElement_t enumeration type in the Sketch. Since AutoConnectElement also acts as a variant of other elements, it can be applied to handle elements collectively. At that time, the type can be referred to by the typeOf() function. The following example changes the font color of all AutoConnectText elements of a custom Web page to gray.
AutoConnectAux customPage;
AutoConnectElementVT& elements = customPage.getElements();
for (AutoConnectElement& elm : elements) {
if (elm.typeOf() == AC_Text) {
AutoConnectText& text = reinterpret_cast<AutoConnectText&>(elm);
text.style = "color:gray;";
}
}
The enumerators for ACElement_t are as follows:
- AutoConnectButton: AC_Button
- AutoConnectCheckbox: AC_Checkbox
- AutoConnectElement: AC_Element
- AutoConnectFile: AC_File
- AutoConnectInput: AC_Input
- AutoConnectRadio: AC_Radio
- AutoConnectRange: AC_Range
- AutoConnectSelect: AC_Select
- AutoConnectStyle: AC_Style
- AutoConnectSubmit: AC_Submit
- AutoConnectText: AC_Text
- Uninitialized element: AC_Unknown
Furthermore, to convert an entity that is not an AutoConnectElement to its native type, you must re-interpret that type with c++. Or, you can be coding the Sketch more easily with using the as<T> function.
AutoConnectAux customPage;
AutoConnectElementVT& elements = customPage.getElements();
for (AutoConnectElement& elm : elements) {
if (elm.type() == AC_Text) {
AutoConnectText& text = customPage[elm.name].as<AutoConnectText>();
text.style = "color:gray;";
// Or, it is also possible to write the code further reduced as follows.
// customPage[elm.name].as<AutoConnectText>().style = "color:gray;";
}
}
AutoConnectButton¶
AutoConnectButton generates an HTML <button type="button">
tag and locates a clickable button to a custom Web page. Currently AutoConnectButton corresponds only to name, value, an onclick attribute of HTML button tag. An onclick attribute is generated from an action
member variable of the AutoConnectButton, which is mostly used with a JavaScript to activate a script.
Sample
AutoConnectButton button("button", "OK", "myFunction()");
On the page:
Constructor¶
AutoConnectButton(const char* name, const char* value, const String& action, const ACPosterior_t post)
name¶
It is the name
of the AutoConnectButton element and matches the name attribute of the button tag. It also becomes the parameter name of the query string when submitted.
value¶
It becomes a value of the value
attribute of an HTML button tag.
action¶
action is String data type and is an onclick attribute fire on a mouse click on the element. It is mostly used with a JavaScript to activate a script.1 For example, the following code defines a custom Web page that copies a content of Text1
to Text2
by clicking Button
.
const char* scCopyText = R"(
<script>
function CopyText() {
document.getElementById("Text2").value = document.getElementById("Text1").value;
}
</script>
)";
ACInput(Text1, "Text1");
ACInput(Text2, "Text2");
ACButton(Button, "COPY", "CopyText()");
ACElement(TextCopy, scCopyText);
post¶
Specifies a tag to add behind the HTML code generated from the element. The default values is AC_Tag_None
.
AutoConnectCheckbox¶
AutoConnectCheckbox generates an HTML <input type="checkbox">
tag and a <label>
tag. It places horizontally on a custom Web page by default.
Sample
AutoConnectCheckbox checkbox("checkbox", "uniqueapid", "Use APID unique", false);
On the page:
Constructor¶
AutoConnectCheckbox(const char* name, const char* value, const char* label, const bool checked, const ACPosition_t labelPosition, const ACPosterior_t post)
name¶
It is the name
of the AutoConnectCheckbox element and matches the name attribute of the input tag. It also becomes the parameter name of the query string when submitted.
value¶
It becomes a value of the value
attribute of an HTML <input type="checkbox">
tag.
label¶
A label is an optional string. A label is always arranged on the right side of the checkbox. Specification of a label will generate an HTML <label>
tag with an id
attribute. The checkbox and the label are connected by the id attribute.
Only will be displayed if a label is not specified.
checked¶
A checked is a Boolean value and indicates the checked status of the checkbox. The value of the checked checkbox element is packed in the query string and sent.
labelPosition¶
The position of the label belonging to the checkbox can be specified around the element. The labelPosition specifies the position of the label to generate with ACPostion_t enumeration value. The default value is AC_Behind
.
AC_Infront
: Place a label in front of the check box.AC_Behind
: Place a label behind the check box.
post¶
Specifies a tag to add behind the HTML code generated from the element. The default values is AC_Tag_BR
.
AutoConnectFile¶
AutoConnectFile generates an HTML <input type="file">
tag and a <label>
tag. AutoConnectFile enables file upload from the client through the web browser to ESP8266/ESP32 module. You can select the flash in the module, external SD device or any output destination as the storage of the uploaded file.
Sample
AutoConnectFile file("file", "", "Upload:", AC_File_FS)
On the page:
Constructor¶
AutoConnectFile(const char* name, const char* value, const char* label, const ACFile_t store, const ACPosterior_t post)
name¶
It is the name
of the AutoConnectFile element and matches the name attribute of the input tag. It also becomes the parameter name of the query string when submitted.
value¶
File name to be upload. The value contains the value entered by the client browser to the <input type="file">
tag and is read-only. Even If you give a value to the constructor, it does not affect as an initial value like a default file name.
label¶
A label
is an optional string. A label is always arranged on the left side of the input box. Specification of a label will generate an HTML <label>
tag with an id attribute. The input box and the label are connected by the id attribute.
store¶
Specifies the destination to save the uploaded file. The destination can be specified the following values in the ACFile_t enumeration type.
AC_File_FS
: Save as the SPIFFS file in flash of ESP8266/ESP32 module.AC_File_SD
: Save to an external SD device connected to ESP8266/ESP32 module.AC_File_Extern
: Pass the content of the uploaded file to the uploader which is declared by the Sketch individually. Its uploader must inherit AutoConnectUploadHandler class and implements _open, _write and _close function.
Built-in uploader is ready.
AutoConnect already equips the built-in uploader for saving to the SPIFFS as AC_File_FS and the external SD as AC_File_SD. It is already implemented inside AutoConnect and will store uploaded file automatically.
post¶
Specifies a tag to add behind the HTML code generated from the element. The default values is AC_Tag_BR
.
AutoConnectInput¶
AutoConnectInput generates an HTML <input type="text">
, <input type="number">
or <input type="password">
tag and a <label>
tag. It can also have a placeholder. The value of the input box is passed to the destination in the query string and can be retrieved programmatically. You can also update from the Sketches.
Sample
AutoConnectInput input("input", "", "Server", "MQTT broker server");
On the page:
Constructor¶
AutoConnectInput(const char* name, const char* value, const char* label, const char* pattern, const char* placeholder, const ACPosterior_t post, const ACInput_t apply)
name¶
It is the name
of the AutoConnectInput element and matches the name attribute, the id attribute of the input tag. It also becomes the parameter name of the query string when submitted.
value¶
It becomes a string value of the value
attribute of an HTML <input type="text">
tag. The text entered from the custom Web page will be grouped in the query string of the form submission and the string set before accessing the page will be displayed as the initial value.
label¶
A label
is an optional string. A label is always arranged on the left side of the input box. Specification of a label will generate an HTML <label>
tag with an id attribute. The input box and the label are connected by the id attribute.
pattern¶
A pattern
specifies a regular expression that the AutoConnectInput element's value is checked against on form submission. If it is invalid, the background color will change, but it will be sent even if the data format does not match. To check whether the entered value matches the pattern, use the isValid function.
- The password that must contain 8 or more characters that are of at least one number, and one uppercase and lowercase letter:
(?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{8,}
-
Email address as characters@characters.domain:
[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,}
-
IP address:
(([0-9]|[1-9][0-9]|1[0-9]{2}|2[0-4][0-9]|25[0-5])\\.){3}([0-9]|[1-9][0-9]|1[0-9]{2}|2[0-4][0-9]|25[0-5])
-
Host name of Internet:
(([a-zA-Z0-9]|[a-zA-Z0-9][a-zA-Z0-9\-]*[a-zA-Z0-9])\.)*([A-Za-z0-9]|[A-Za-z0-9][A-Za-z0-9\-]*[A-Za-z0-9])
-
Date (MM/DD/YYYY) as range 1900-2099:
(0[1-9]|1[012])[- \/.](0[1-9]|[12][0-9]|3[01])[- \/.](19|20)\d\d
-
Twitter account:
^@?(\w){1,15}$
placeholder¶
A placeholder is an option string. Specification of a placeholder will generate a placeholder
attribute for the input tag.
post¶
Specifies a tag to add behind the HTML code generated from the element. The default values is AC_Tag_BR
.
apply¶
Specifies the type of input that the text box accepts. AutoConnectInput will generate either a input type="text"
, input type="password"
, or input type="number"
tag based on the apply
specifying as input type. The input type can be specified the following values in the ACInput_t enumeration type.
AC_Input_Text
:input type="text"
AC_Input_Password
:input type="password"
AC_Input_Number
:input type="number"
Numerical keypad is different
When the AutoConnectInput element with the AC_Input_Number
applied is focused on the browser, the numeric keypad may be displayed automatically. For popular mobile OSes such as Android and iOS, the numeric keypad has the following styles and is different with each OS.
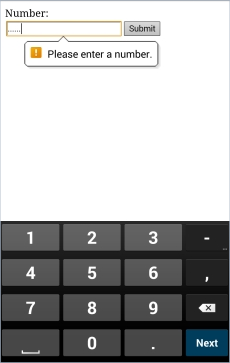
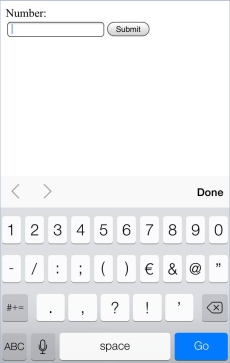
AutoConnectRadio¶
AutoConnectRadio generates several HTML <input type="radio">
tags grouped together. It also assigns an equal number of <label>
tags to each <input type="radio">
tag and stores the values of the choices that make up a radio button as a String collection.
Sample
AutoConnectRadio radio("radio", { "30 sec.", "60 sec.", "180 sec." }, "Update period", AC_Vertical, 1);
On the page:
Constructor¶
AutoConnectRadio(const char* name, std::vector<String> const& values, const char* label, const ACArrange_t order, const uint8_t checked, const ACPosterior_t post)
name¶
It is the name of the AutoConnectRadio element, which matches the name attribute of the input tag and defines the radio group by this name. It is also the name
parameter in the query string during submission.
values¶
A group of radio buttons is a set of <input type="radio">
tags with the same name; AutoConnectRadio defines a radio group based on an array of Strings specified as values. A values
is an array of String, actually a std::vector. The sketch can allocate its String array using std::vector's List-initialization.
label¶
A label is an optional string. A label will be arranged in the left or top of the radio buttons according to the order. Specification of a label will generate an HTML <label>
tag with an id
attribute. The radio buttons and the label are connected by the id attribute.
order¶
A order
specifies the direction to arrange the radio buttons. It is a value of type ACArrange_t
and accepts one of the following:
AC_Horizontal
: Horizontal arrangement.AC_Vertical
: Vertical arrangement.
A label will place in the left or the top according to the order.
checked¶
A checked
specifies the index number (1-based) of the values to be checked. If this parameter is not specified neither item is checked.
post¶
Specifies a tag to add behind the HTML code generated from the element. The default values is AC_Tag_BR
.
AutoConnectRange¶
AutoConnectRange generates an HTML <input type="range">
tag and a <label>
tag.
Sample
AutoConnectRange range("bri", 0, "Brightness", -2, 2, 1, AC_Infront);
On the page:
Constructor¶
AutoConnectRange(const char* name, const int value, const char* label, const int min, const int max, const int step, const ACPosition_t magnify, const ACPosterior_t post, const char* style)
name¶
It is the name
of the AutoConnectRange element and matches the name attribute, the id attribute of the input tag. It also becomes the parameter name of the query string when submitted.
value¶
It becomes a string value of the value
attribute of an HTML <input type="range">
tag, which indicates the default value of the range.
label¶
A label
is an optional string. A label is always arranged on the left side of the range slider. Specification of a label will generate an HTML <label>
tag with an id attribute. The range slider and the label are connected by the id attribute.
min¶
Specifies the most negative value within the range of allowed values and must not be less than the value
argument.
max¶
It defines the greatest value in the range of permitted values.
step¶
It is a number that specifies the granularity that the value must adhere to. The default is 1. As you move the slider, it increases or decreases the value according to the step
in granularity.
magnify¶
Displays the current value of the range on the left or right side of the slider.
AC_Infront
: Displays the current value on the left side.AC_Behind
: Displays the current value on the right side.AC_Void
: No display the current value. This is the default.
post¶
Specifies a tag to add behind the HTML code generated from the element. The default values is AC_Tag_BR
.
style¶
A style
specifies the qualification style to give to the content and can use the style attribute format as it is.
AutoConnectStyle¶
AutoConnectStyle inserts the string given by the value into the style block of a custom Web page as it is raw.
The validity as CSS will not be checked
AutoConnectStyle does not do syntax checking and semantic analysis of value. Insert the specified string into the style block of the custom Web page without processing it. Therefore, specifying the wrong CSS will modulate the behavior of the custom Web page.
Constructor¶
AutoConnectStyle(const char* name, const char* value)
name¶
It is the name
of the AutoConnectStyle element and is useful only to access this element from the Sketch. It does not affect the generated HTML code.
value¶
The raw CSS code. It is not necessary to write <style>
</style>
tags.
AutoConnectSelect¶
AutoConnectSelect generates an HTML <select>
tag (drop-down list) and few <option>
tags.
Sample
AutoConnectSelect select("select", { String("Europe/London"), String("Europe/Berlin"), String("Europe/Helsinki"), String("Europe/Moscow"), String("Asia/Dubai") }, "Select TZ name");
On the page:
Constructor¶
AutoConnectSelect(const char* name, std::vector<String> const& options, const char* label, const uint8_t selected, const ACPosterior_t post)
name¶
It is the name
of the AutoConnectSelect element and matches the name attribute of the select tags.
options¶
An options
is an array of String type for the options which as actually std::vector for an HTML <option>
tag. It is an initialization list can be used. The option tags will be generated from each entry in the options, the amount of which is the same as the number of items in an options
.
label¶
A label
is an optional string. A label is always arranged on the left side of the drop-down list. Specification of a label will generate an HTML <label>
tag with an id attribute. The select tag and the label are connected by the id attribute.
selected¶
A selected
is an optional value. Specifies that an option should be pre-selected when the page loads.
post¶
Specifies a tag to add behind the HTML code generated from the element. The default values is AC_Tag_BR
.
AutoConnectSubmit¶
AutoConnectSubmit generates an HTML <input type="button">
tag attached onclick
attribute. The native code of the onclick
attribute is the submission of the form with the POST method.
Sample
AutoConnectSubmit submit("submit", "Save", "/mqtt_save");
On the page:
Constructor¶
AutoConnectSubmit(const char* name, const char* value, const char* uri, const ACPosterior_t post)
name¶
It is the name
of the AutoConnectSubmit element and matches the name attribute of the input tag.
value¶
It becomes a string of the value
attribute of an HTML <input type="button">
tag. The value
will be displayed as a label of the button.
uri¶
A uri
specifies the URI to send form data when the button declared by AutoConnectSubmit is clicked.
The query string of the form data sent with AutoConnectSubmit contains the URI of the page. Its parameter name is _acuri
. In Sketch, you can know the called URI by referring to the _acuri
parameter with the destination page handler. The actual query string is as follows:
_acuri=CALLER_URI
post¶
Specifies a tag to add behind the HTML code generated from the element. The default values is AC_Tag_None
.
AutoConnectText¶
AutoConnectText generates a text content enclosed in <div>
, <p>
or <span>
tags; if the style parameter is provided, the style attributes is added. A kind of HTML tag applied depends on the value of the post parameter as follows:
- AC_Tag_None:
<span id='name' style='style'>value</span>
- AC_Tag_BR:
<span id='name' style='style'>value</span><br>
- AC_Tag_P:
<p id='name' style='style'>value</p>
- AC_Tag_DIV:
<div id='name' style='style'>value</div>
Sample
AutoConnectText text("text", "Publishing the WiFi signal strength to MQTT channel. RSSI value of ESP8266 to the channel created on ThingSpeak", "font-family:serif;color:#4682b4;");
On the page:
Constructor¶
AutoConnectText(const char* name, const char* value, const char* style, const char* format, const ACPosterior_t post)
name¶
A name
does not exist in the generated HTML. It provides only a means of accessing elements with the Sketches.
value¶
It becomes content and also can contain the native HTML code, but remember that your written code is enclosed by the div tag.
style¶
A style
specifies the qualification style to give to the content and can use the style attribute format as it is.
format¶
A format
is a pointer to a null-terminated multi byte string specifying how to interpret the value. It specifies the conversion format when outputting values. The format string conforms to C-style printf library functions, but depends on the Espressif's SDK implementation. The conversion specification is valid only in %s format. (Left and Right justification, width are also valid.)
post¶
Specifies an HTML element that completes the text content. AutoConnectText's post parameter does not specify any behind-supplements, unlike when applied to other elements. A kind of HTML tag applied depends on the enumerated value of the post parameter as follows:
- AC_Tag_None:
<span id='name' style='style'>value</span>
- AC_Tag_BR:
<span id='name' style='style'>value</span><br>
- AC_Tag_P:
<p id='name' style='style'>value</p>
- AC_Tag_DIV:
<div id='name' style='style'>value</div>
How to coding for the elements¶
Declaration for the elements in Sketches¶
Variables of each AutoConnetElement can be declared with macros. By using the macros, you can treat element name that is String type as variable in sketches.2
ACElement ( name [ , value ] [ , AC_Tag_None | AC_Tag_BR | AC_Tag_P | AC_Tag_DIV ] )
ACButton ( name [ , value ] [ , action ] [ , AC_Tag_None | AC_Tag_BR | AC_Tag_P | AC_Tag_DIV ] )
ACCheckbox ( name [ , value ] [ , label ] [ , true | false ] [ , AC_Infront | AC_Behind ] [ , AC_Tag_None | AC_Tag_BR | AC_Tag_P | AC_Tag_DIV ] )
ACFile ( name [ , value ] [ , label ] [ , AC_File_FS | AC_File_SD | AC_File_Extern ] [ , AC_Tag_None | AC_Tag_BR | AC_Tag_P | AC_Tag_DIV ] )
ACInput ( name [ , value ] [ , label ] [ , pattern ] [ , placeholder ] [ , AC_Tag_None | AC_Tag_BR | AC_Tag_P | AC_Tag_DIV ] [ , AC_Input_Text | AC_Input_Password | AC_Input_Number ])
ACRadio ( name [ , values ] [ , label ] [ , AC_Horizontal | AC_Vertical ] [ , checked ] [ , AC_Tag_None | AC_Tag_BR | AC_Tag_P | AC_Tag_DIV ] )
ACRange ( name [ , value ] [ , label ] [ , min ] [ , max ] [ , step ] [ , AC_Infront | AC_Behind | AC_Void ] [ , AC_Tag_None | AC_Tag_BR | AC_Tag_P | AC_Tag_DIV ] [ , style ] )
ACSelect ( name [ , options ] [ , label ] [ , AC_Tag_None | AC_Tag_BR | AC_Tag_P | AC_Tag_DIV ] )
ACStyle ( name [ , value ] )
ACSubmit ( name [ , value ] [ , uri ] [ , AC_Tag_None | AC_Tag_BR | AC_Tag_P | AC_Tag_DIV ] )
ACText ( name [ , value ] [ , style ] [ , format ] [ , AC_Tag_None | AC_Tag_BR | AC_Tag_P | AC_Tag_DIV ] )
Declaration macro usage
For example, AutoConnectText can be declared using macros.
AutoConnectText caption("caption", "hello, world", "color:blue;")
ACText(caption, "hello, world", "color:blue;")
Variant for AutoConnectElements¶
Some AutoConnectAux APIs specify AutoConnectElements as an argument. There are also functions that return a pointer to AutoConnectElements. AutoConnectElement behaves as a variant type of each element class to make these interfaces a single. Use reinterpret_cast to cast from a variant pointer to an Actual type pointer of AutoConnectElements.
AutoConnectAux aux;
ACText(Text1, "hello, world");
aux.add(Text1);
AutoConnectText* text_p = reinterpret_cast<AutoConnectText*>(aux.getElement("Text1"));
AutoConnectText& text = aux.getElement<AutoConnectText>("Text1");